I believe for every Object-oriented programming (OOP) language, the chore of creating your accessor/mutator (also known as getters/setters) methods for every class that you declared is dreaded by every developer.
public class FooBar {
private String foo;
/*
Accessor Methods
*/
public String getFoo() {
return foo;
}
/*
Mutator Methods
*/
public void setFoo(String foo) {
this.foo = foo;
}
}
With the Lombok library (https://projectlombok.org/), you can speed up your development speed with just annotations!
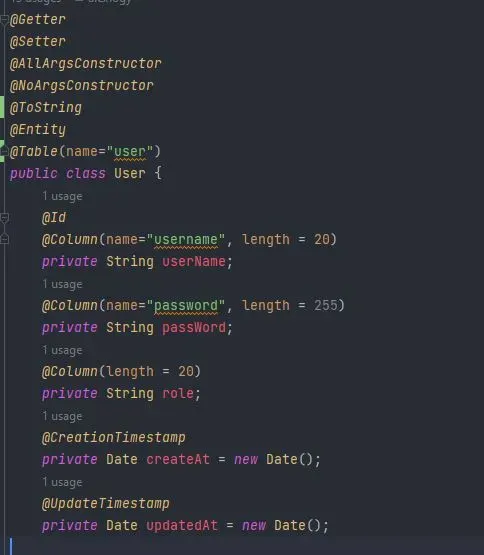
We can create our accessor/mutator methods with just the @Getter and @Setter annotations. That's not all, we can even create our default constructor and parameterized constructors with just the @NoArgsConstructor and @AllArgsConstructor annotations.
Ever faced a problem where you have to create your toString() function to print your object? Fear not! The @ToString annotation will automatically create it for you.
This library have assisted me tremendously in speeding up my development speed since I known of it's existence. Try it today!